Introduction
Welcome to the Vauld Enterprise API's!
You can use our API to access our secure endpoints, which can help you create wallets for your users or create lending and borrowing orders without having to create a user/wallet. Welcome to the Vauld Enterprise API documentation! You can use our API to access secure endpoints, which can help you create wallets for your users and get access to our lending and borrowing functionalities. We have language bindings in JavaScript. You can view relevant code samples over to the right in the dedicated dark section as shown. You can switch the programming language of the examples shown with tabs displayed on the top-left corner of the region.
You can use our API to access our secure endpoints, which can help you create wallets for your users or create lending and borrowing orders without having to create a user/wallet. Welcome to the Vauld Enterprise API documentation! You can use our API to access secure endpoints, which can help you create wallets for your users and get access to our lending and borrowing functionalities. We have language bindings in JavaScript. You can view relevant code samples over to the right in the dedicated dark section as shown. You can switch the programming language of the examples shown with tabs displayed on the top-left corner of the region.
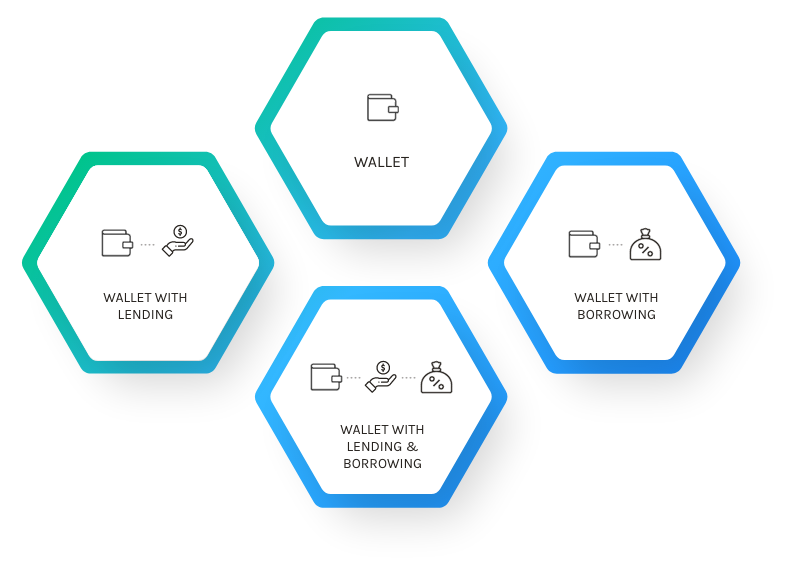
Getting Started
Base URL for testnet
https://apis.bankofhodlers.com
To get access to production URL's and API keys, contact us at [email protected]. The testnet we use for ETH and ERC20 is kovan network.
For more reading on mainnets and testnets: Ethereum 101: Mainnet and testnets
Alright, if that's all good, let's get started!
Authentication
We use HMAC based authentication.
To authenticate the APIs, we create an HMAC hash for the payload (in URI format) using the SHA-256 hashing algorithm using the provided APIKey as the seed. The HMAC hash generated is converted to it's Hex notation and is passed to the response body in the format mentioned below, with the headers set to
To authenticate the APIs, we create an HMAC hash for the payload (in URI format) using the SHA-256 hashing algorithm using the provided APIKey as the seed. The HMAC hash generated is converted to it's Hex notation and is passed to the response body in the format mentioned below, with the headers set to
Content-Type: application/json.
hmac: _HMAC_Hash_asHex_
To authenticate, use this format:
# You can use any hmac library that supports SHA256 algorithm
# Illustrative code generate hmac for a payload
const CryptoJS = require('CryptoJS');
const jsonToURI = async (jsonObj) => {
var output = '';
var keys = Object.keys(jsonObj);
keys.forEach(function(key) {
output = output + key + '=' + jsonObj[key] + '&';
});
return output.slice(0, -1);
}
const payload = '_Request_inJSON_';
const secret = '_your_APIKey_' // make this your secret!!
const URIencodedPayload = jsonToURI(JSON.parse(payload));
const hmac = CryptoJS.HmacSHA256(URIencodedPayload, secret);
const hash = hmac.toString(CryptoJS.enc.Hex); //_HMAC_Hash_asHex_
// Add custom values to request header, e.g.
request.headers.add({
key: 'Content-Type',
value: 'application/json'
});
request.headers.add({
key: 'hmac',
value: hash
});
Introduction - Fiat <> Crypto Trading APIs
These set of APIs are for enterprises looking to integrate FIAT rails in India. They provide the ability to create an INR wallet for users, deposit INR to the wallet, and buy and sell cryptocurrencies with the deposited INR amount. They allow users to deposit INR via Cards/UPI/NEFT/IMPS/RTGS, and to withdraw INR to any bank account in 1 business day.
This document outlines the details of the APIs used to:
1. Create a user account
2. Complete KYC for a user
3. Deposit and withdraw INR
4. Buy and sell crypto with INR
5. Withdraw Crypto
NOTE: Prerequisites for using the APIs
1. Contact [email protected] for your API_KEY and orgID
2. Creating a user account is a mandatory prerequisite for all the other APIs
3. Completing their KYC is a mandatory prerequisite for INR deposits/withdrawals and INR Buy/Sell
Base URL:
This document outlines the details of the APIs used to:
1. Create a user account
2. Complete KYC for a user
3. Deposit and withdraw INR
4. Buy and sell crypto with INR
5. Withdraw Crypto
NOTE: Prerequisites for using the APIs
1. Contact [email protected] for your API_KEY and orgID
2. Creating a user account is a mandatory prerequisite for all the other APIs
3. Completing their KYC is a mandatory prerequisite for INR deposits/withdrawals and INR Buy/Sell
Base URL:
https://api-dev.bankofhodlers.com/enterprise/api/v1/
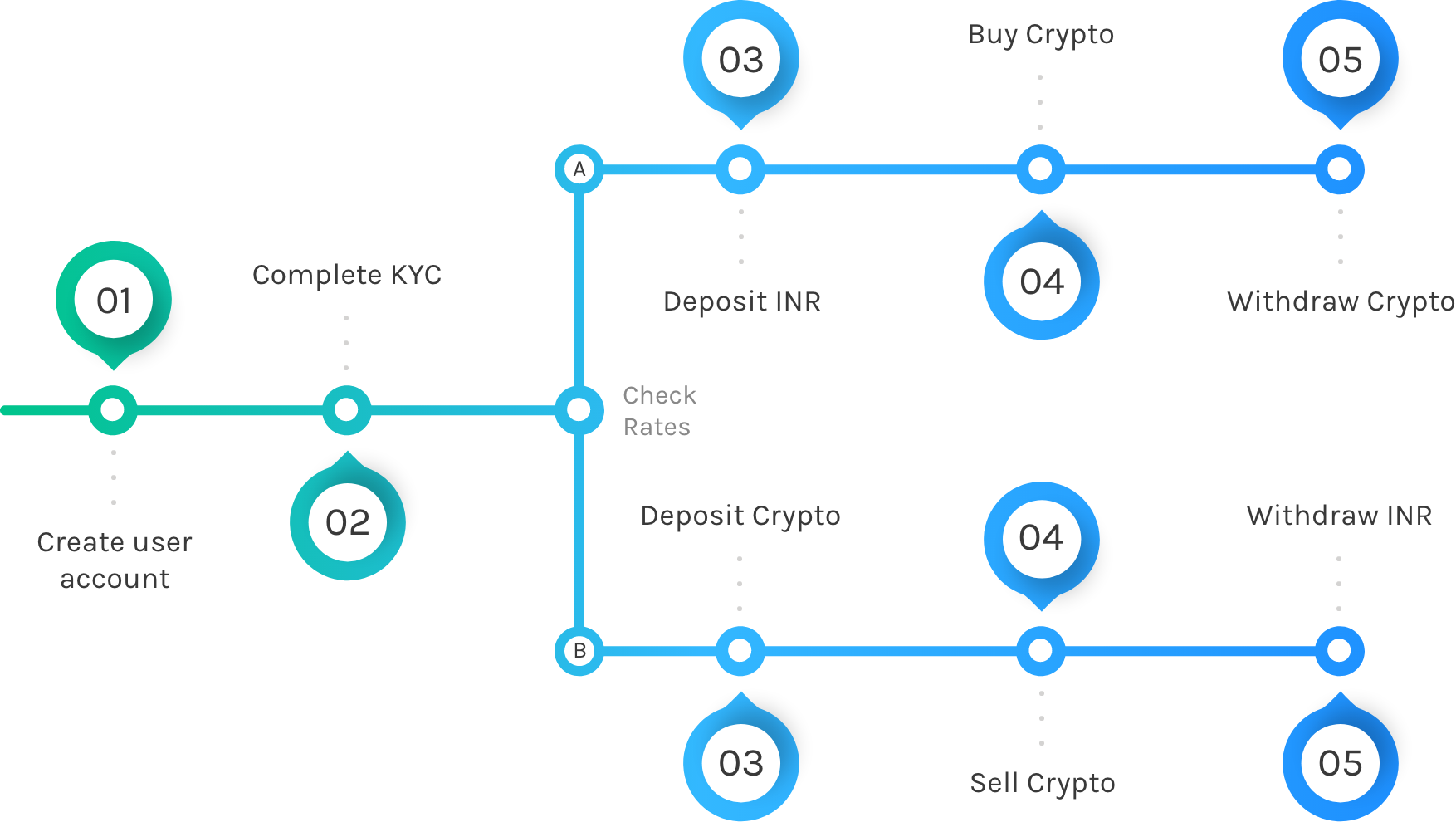
Note:
1. All token values in calls and responses will be in base value.
Token | Symbol | Base |
---|---|---|
Bitcoin | btc | 100000000 |
Ethereum | eth | 1000000000000000000 |
Ripple | xrp | 1000000 |
Stellar | xlm | 10000000 |
True USD | tusd | 1000000000000000000 |
Multi-Collateral Dai | dai | 1000000000000000000 |
Tether | usdt | 1000000 |
USD Coin | usdc | 1000000 |
Basic Attention Token | bat | 1000000000000000000 |
Paxos | pax | 1000000000000000000 |
2. All KYC documents are auto-approved in 30 seconds without verification in the test environment
3. INR Deposits confirmations are auto-approved without verification. INR withdrawals will remain in pending state.
4. For ETH and ERC20 tokens transaction, kovan network is used in testnet.
5. We dont prefill crypto wallets with any tokens, so please transfer testnet funds to your assigned wallet address for smooth exchange to and from fiat.
6. Fee Structure
Token | Withdrawal | Deposit | Exchange Fees |
---|---|---|---|
BTC | 0.0004 BTC | 0 | 0.25 |
ETH | 0.003 ETH | 0 | 0.25 |
TUSD | 0.6 TUSD | 0 | 0.25 |
DAI | 0.6 DAI | 0 | 0.25 |
PAX | 0.6 PAX | 0 | 0.25 |
USDT | 0.6 USDT | 0 | 0.25 |
USDC | 0.6 USDC | 0 | 0.25 |
XRP | 0.6 XRP | 0 | 0.25 |
XLM | 0.01 XLM | 0 | 0.25 |
BAT | 2 BAT | 0 | 0.25 |
INR | 0 | 0 | 0.25 |
7. Minimum amount for deposit to reflect is $1 worth of token being deposited.
8. Minimum amount for withdrawal is $5 worth of token being withdrawn.
9. Minimum amount to exchange is at least $10 worth of token being exchanged.
10. All responses will have the following format
Sample response format : Success
Sample response format : Failed
In the documentation below, only the data part of the response will be mentioned.
User Module
Creating user account
HTTP POST Request
{{url}}/users/create
Request Parameters
Parameter | comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
userIdentifier | A unique identifier of the user. Can be alphanumeric value. Will be used to identify a user from your end |
isCorporate | For KYC purposes (Corporate users) true/false |
Response Data
Parameter | comments |
---|---|
success | true or false based on whether user creation succeeded or failed |
userID | The userID returned while creating the user |
Get User Details
HTTP POST Request
{{url}}/users/details
Request Parameters
Parameter | comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
userID | The userID returned while creating the user |
Response Data
Parameter | Value | Comments |
---|---|---|
userID | The ID of the user who created the request | |
kycStatus |
{ status: failed, cause:”invalid document” } |
There can be 5 status: 1. success 2. open 3. failed 4. pending 5. notSubmitted If failed, cause will also be mentioned Status is open when KYC basic is submitted but Documents are not uploaded |
inrBalance | {balance:amount, locked: amount} | |
crypto | [{token: “btc”, address:”btcaddr”, balance:”value”, locked:”value”}] | 'balance' and 'locked' is in base value, for Eg: 1 ETH will be 1000000000000000000 |
depositBankDetails | {name:”name”, accountNumber:”accNo”, ifsc:”ifsc”, "accountType": "current", "accountConfig": "centralAccount"} | Account to which the user should deposit INR to be reflected in his account. accountType can be 'current' or 'savings' accountConfig can be 'centralAccount' or 'van'. 'van' is unique virtual account number given to each user where no confirmations are needed post deposit. For 'centralAccount' after deposits are made, transaction id/reference id of deposits needs to be submitted at '/fiat/paymentConfirmed' endpoint. |
withdrawBankDetails | [{accountID:”id”, name:”name”, accountNumber:”accNo”, ifsc:”ifsc”}] | Array of all accounts added by the user |
Get All Transactions
HTTP POST Request
{{url}}/users/txns
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
userID | The userID returned while creating the user |
Response Data
Parameter | Comments |
---|---|
txns | Array of all transactions by the user |
Get Organisation user list
HTTP POST Request
{{url}}/users/orgUsers
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
Response Data
Parameter | Comments |
---|---|
allOrgUsers |
Array of all users under the organisation
Sample Response: { "success": true, "data": { "allOrgUsers": [ { "userID": "5fb6b1b9643b3500180q409d", "userIdentifier": "test1911" } ] } } |
Individual KYC Module
Complete KYC
The APIs below outline an instant, automated KYC verification process for your users. They will enable you to initiate the KYC process, upload the necessary documents, verify them instantly and complete the KYC process.
The prerequisites for this KYC process are:
1. A valid Indian identity document (Aadhar Card, Voter ID or Passport). NOTE: We do not support driving licenses at the moment.
2. A PAN card
3. A clear selfie
The prerequisites for this KYC process are:
1. A valid Indian identity document (Aadhar Card, Voter ID or Passport). NOTE: We do not support driving licenses at the moment.
2. A PAN card
3. A clear selfie
Initiate KYC verification process
HTTP POST Request
{{url}}/kyc/initiateKyc
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
userID | The userID returned while creating the user |
docType | User identification document that will be submitted for verification. Following documents are supported 1. passport 2. voterID 3. aadhar |
Response Data
Parameter | Value | Comments |
---|---|---|
success | true/false |
Request for upload url
HTTP POST Request
{{url}}/kyc/getUploadUrl
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
userID | The userID returned while creating the user |
Response Data
Parameter | Comments |
---|---|
documentFront | URL to front side of selected document while initiating kyc process [passport/voterID/aadhar] |
documentBack | URL to back side of selected document while initiating kyc process [passport/voterID/aadhar] |
selfie | URL to upload selfie image of the user |
panCard | URL to upload PAN Card of the user |
Upload images
HTTP PUT Request
1. Upload the images to the url received from the previous step using a PUT request.
2. It is an amazon S3 bucket URL which expires in 30 minutes
3. Image type should be JPG/JPEG and file size should be a maximum of 5 MB
2. It is an amazon S3 bucket URL which expires in 30 minutes
3. Image type should be JPG/JPEG and file size should be a maximum of 5 MB
Verify Document
- This endpoint needs to be called for all the uploaded document URLs [ i.e for front/back side of the selected document [passport/voterID/aadhar] and PAN Card.]
- This endpoint helps in real time document validation.
-
For example, if passport was selected, this endpoint needs to be called 3 times with following parameter:
- doc: passport, frontOrBack: front
- doc: passport, frontOrBack: back
- doc: pan, frontOrBack: front
HTTP POST Request
{{url}}/kyc/verifyDoc
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
userID | The userID returned while creating the user |
documentType | Document type that needs to be verified. passport/aadhar/voterID/pan |
frontOrBack | front / back , depending on which side of the document needs to be verified. |
Response Data
Parameter | Comments |
---|---|
data | Data that was machine read from the uploaded document. |
Verify Selfie
HTTP POST Request
{{url}}/kyc/verifySelfie
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
userID | The userID returned while creating the user |
Response Data
Parameter | Comments |
---|---|
data | Data that was machine read from the uploaded document. |
Instant KYC Approval
HTTP POST Request
{{url}}/kyc/instantApproval
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
userID | The userID returned while creating the user |
Response Data
Parameter | Comments |
---|---|
success | true/false |
Manual KYC verification flow
HTTP POST Request
{{url}}/kyc/initiateManualVerification
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
userID | The userID returned while creating the user |
Response Data
Parameter | Comments |
---|---|
success | true or false |
KYC Status
HTTP POST Request
{{url}}/kyc/getKYCStatus
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
userID | The userID returned while creating the user |
Response Data
Parameter | Comments |
---|---|
status |
There can be 5 status
|
Corporate KYC
Complete KYC
The APIs below outline the KYC verification process for your Corporate users. They will enable you to initiate the Corporate KYC process, upload the necessary documents and submit them for verification with Vauld.
The prerequisites for this Corporate KYC process are:
1. A valid Nation identity document of the Director(s) of the company, upto 4 Directors can be uploaded/verified - images (front and back)
2. Company incorporation certificate - pdf
3. Directors equity split document - pdf
4. Directors resolution document - pdf
5. Fund Source document - pdf
The prerequisites for this Corporate KYC process are:
1. A valid Nation identity document of the Director(s) of the company, upto 4 Directors can be uploaded/verified - images (front and back)
2. Company incorporation certificate - pdf
3. Directors equity split document - pdf
4. Directors resolution document - pdf
5. Fund Source document - pdf
Initiate Corporate KYC verification process
HTTP POST Request
{{url}}/kyc/initiateCorporateKYC
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
userID | The userID returned while creating the user |
Response Data
Parameter | Value | Comments |
---|---|---|
success | true/false |
Request for upload url
HTTP POST Request
{{url}}/kyc/getUploadURL
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
userID | The userID returned while creating the user |
Response Data
Parameter | Comments |
---|---|
nationalIDDirector1Front | URL to upload front side of selected document while initiating kyc process Document type expected: Image |
nationalIDDirector1Back | URL to upload back side of selected document while initiating kyc process Document type expected: Image |
nationalIDDirector2Front | URL to upload front side of selected document while initiating kyc process Document type expected: Image |
nationalIDDirector2Back | URL to upload back side of selected document while initiating kyc process Document type expected: Image |
nationalIDDirector3Front | URL to upload front side of selected document while initiating kyc process Document type expected: Image |
nationalIDDirector3Back | URL to upload back side of selected document while initiating kyc process Document type expected: Image |
nationalIDDirector4Front | URL to upload front side of selected document while initiating kyc process Document type expected: Image |
nationalIDDirector4Back | URL to upload back side of selected document while initiating kyc process Document type expected: Image |
incorporationCert | URL to upload Company incorporation certificate Document type expected: PDF |
dirEquitySplit | URL to upload Directors equity split document Document type expected: PDF |
dirResolution | URL to upload Directors resolution document Document type expected: PDF |
fundSource | URL to upload Source of Funds document Document type expected: PDF |
Upload images/documents
HTTP PUT Request
- Upload the image/document to the URL received from the previous step via a PUT request.
- The URL is signed and will expire in 30 minutes.
- In case of an event of a URL expiring, Please do generate a new URL via the previous step.
- In the case of an event of a URL expiring, documents successfully uploaded would be not required to be reuploaded.
- Images should be in the JPG/JPEG format.
- Documents should be in the PDF.
- File sizes should not exceed a maximum of 5 MB
- Upload the image/document to the URL received from the previous step via a PUT request.
Submit Corporate KYC
- This endpoint needs to be called so that Vauld can verify the Corporate KYC of a user once all the documents are submitted
HTTP POST Request
{{url}}/kyc/submitCorporateKYC
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
userID | The userID returned while creating the user |
Response Data
Parameter | Comments |
---|---|
data | Data that was machine read from the uploaded document. |
Deposit/Withdraw Module
Integrate Instant Payment SDK (Deprecated)
Add this script in the head section of your index.html
<script type="text/javascript" src="https://bohstaticfiles.s3.amazonaws.com/cardsSDK_v1_0.min.js"></script>
This SDK is for test environment. You may request us for the production SDK after successfully integrating and testing the functionality.
You can initiate an instant payment by the following function that is exposed via BoH object on the window
window.BoH.makeInstantPayment(paymentRequest, 'paymentEl', successCallbackFn, errorCallbackFn);
Method Parameters
Parameter | Comments |
---|---|
paymentRequest |
A javascript object with the following key value pairs:
The amount passed should be a valid javascript number |
paymentEl | The id of the html DOM element (preferably a full width div element) in which the payment page would be rendered on the website |
successCallbackFn |
The javascript callback function to be invoked in case of a successful transaction. The response object passed as the argument to the function would be of the format:
|
errorCallbackFn |
The javascript callback function to be invoked in case of a failed transaction. The response object passed as the argument to the function would be of the format:
|
You can cancel an ongoing payment by invoking the following method:
window.BoH.cancelOnGoingPayment();
Submit deposit confirmation
HTTP POST Request
{{url}}/fiat/paymentConfirmed
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
userID | The userID returned while creating the user (NOTE: Completing the users KYC is a mandatory prerequisite for this API) |
paymentID | RTGS/IMPS/NEFT reference id |
amount | Amount in INR transferred for deposits |
Response Data
Parameter | Comments |
---|---|
txnID | Reference ID for submitted deposit entry |
Add withdraw bank account details
HTTP POST Request
{{url}}/fiat/addBankAccount
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
userID | The userID returned while creating the user |
name | Name of account holder |
accountNumber | Account number |
ifsc | IFSC code |
Response Data
Parameter | comments |
---|---|
accountID | ID for the account just added. Pass this along when requesting withdrawal |
status | Approved/Rejected |
error | if rejected, reason for rejection |
Remove withdraw bank account details
HTTP POST Request
{{url}}/fiat/removeBankAccount
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
userID | The userID returned while creating the user |
bankAccountID | ID generated while adding bank account details, Alternative this can be fetched from userDetails response in withdrawBankDetails section. |
Response Data
Parameter | comments |
---|---|
accountDetails | Updated account details array of object |
Request withdrawal
HTTP POST Request
{{url}}/fiat/requestWithdrawal
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
userID | The userID returned while creating the user (NOTE: Completing the users KYC is a mandatory prerequisite for this API) |
bankAccountID | Bank account ID |
amount | Amount in String |
Response Data
Parameter | comments |
---|---|
message | Comments for readability of the transaction |
txnID | Reference ID for submitted withdrawal entry |
Buy/Sell Module
Fetch Price
HTTP POST Request
{{url}}/fiat/getAllPrice
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
Response Data
Parameter | Value | Comments |
---|---|---|
allPair |
|
Current bid and ask for token pairs, 'bid' and 'ask' is in INR |
Get Token Exchange Amount
HTTP POST Request
{{url}}/fiat/getPrice
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
amount | Amount is in base value, Eg: if 1 ETH is required, pass 1000000000000000000 |
type | 'buy'/'sell' |
token | Symbol of token supported by our platform |
Response Data
Parameter | Comments |
---|---|
baseToken | Token symbol which is getting exchanged |
quoteToken | Token symbol to which exchange is desired |
baseAmount | 'baseAmount' is in base value. Eg: 1 ETH will be 1000000000000000000 |
quoteAmount | 'quoteAmount' is in base value. Eg: 1 ETH will be 1000000000000000000 |
exType | Type of exchange |
Create order
HTTP POST Request
{{url}}/fiat/createOrder
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
userID | The userID returned while creating the user |
type | buy/sell |
token | Name of token “btc”, ”eth” etc |
inrAmount | INR value |
tokenAmount | tokenAmount (amount is in base value, e.g if 1 ETH is required, pass 1000000000000000000) |
Response Data
Parameter | Value | Comments |
---|---|---|
orderID | value | Order ID of the order placed. Can be used to track transaction |
Get order status
HTTP POST Request
{{url}}/fiat/details
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
userID | The userID returned while creating the user (NOTE: Completing the users KYC is a mandatory prerequisite for this API) |
orderID | ID of the order placed |
Response Data
Parameter | Value | Comments |
---|---|---|
orderStatus | success/failed/pending | |
type | buy/sell | |
depositToken | btc | Name of token “btc”, ”eth” etc |
destinationToken | inr | Name of token “btc”, ”eth”, "inr" etc |
depositAmount | Deposit token value | |
destinationAmount | Destination token value |
Get all Orders
HTTP POST Request
{{url}}/fiat/allExchangeOrders
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
userID | The userID returned while creating the user |
Response Data
Parameter | Value | Comments |
---|---|---|
txns |
|
status : openOrder , complete, refunded, failed
type : buy, sell depositAmount / destinationAmount : INR token is rupee denominated and crypto amounts are base value ( e.g wei for ETH ) |
Internal INR Transfer
HTTP POST Request
{{url}}/fiat/internalTransfer
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
userID | The userID returned while creating the user |
receiverUserID | The userID of the receiver |
amount | Amount to Send |
token | Please send 'INR'. For sending cryptocurrency use /wallets/send endpoint. |
Response Data
Parameter | Value | Comments |
---|---|---|
txnID | This is the transactionID for debit of token amount from the user's account. |
Webhooks
For events like user creation, balance change,
kyc updates, apart from receiving the details from REST calls,
updates are pushed to the webhook URL as well
for tracking of such updates.
The default template of webhook sent to the requested URL:
{
"event":<eventName>,
"subEvent":<subEventName>,
"eventData":
{
"success":<true/false>,
“data”:
{
… //Relevant data in json format pertaining to event type
}
}
}
Event Types
Event Name | Comments |
---|---|
user | Updates related to users, such as creation of users. |
kyc | Updates related to kyc, such as when kyc is accepted or rejected. |
fiat | Updates related to deposits and withdrawal of fiat currencies |
crypto | Updates related to deposits and withdrawal of crypto tokens. |
SubEvent Types
Event Name | Comments |
---|---|
userCreated | On creation of new user |
status | Change in kyc status |
aadharVerification | Aadhar verification data on kyc verification |
voterIDVerification | VoterID data on kyc verification |
passportVerification | Passport data on kyc verification |
panVerification | PAN data on kyc verification |
selfieVerification | Selfie verification data |
deposit | On deposit of fiat / crypto tokens |
withdrawal | On Withdrawal of fiat / crypto tokens |
internalTransfer | Internal crypto transfer is processed between users of the same organizationID |
Add Webhook URL
HTTP POST Request
{{url}}/misc/addWebhook
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
webhookUrl | URL where webhooks updates can be received via POST call. |
Response Data
Parameter | Value | Comments |
---|---|---|
message | Webhook added successfully | On Successful creation of webhook |
Test Webhook URL
To test if the added URL is properly receiving request
HTTP POST Request
{{url}}/misc/testWebhook
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
Response Data
Parameter | Value | Comments |
---|---|---|
message | Request sent successfully |
On Successful send of testData.
|
Crypto <> Crypto Module
Get Rates for all Pairs
HTTP POST Request
{{url}}/crypto/getAllExchangeRates
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
Response Data
Parameter | Value | Comments |
---|---|---|
allPair |
|
Token before the slash(/) is to receive, i.e. buy, and token after the slash(/) is sell |
Get Rates a single Pair
HTTP POST Request
{{url}}/crypto/getExchangeRate
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
pair | Token pair whose exchange rate is expected |
Response Data
Parameter | Value | Comments |
---|---|---|
pairObject |
|
Token before the slash(/) is to receive, i.e. buy, and token after the slash(/) is sell |
Create order
HTTP POST Request
{{url}}/crypto/createOrder
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
userID | The userID returned while creating the user |
type | buy/sell based on pair convention |
depositToken | Name of token “btc”, ”eth” |
depositAmount | Deposit token amount |
destinationToken | Name of token “btc”, ”eth” |
destinationAmount | Destination token amount |
pairObject | Pair data received from previous step |
Response Data
Parameter | Value | Comments |
---|---|---|
orderID | Order ID of the order placed. Can be used to track transaction |
Get order status
HTTP POST Request
{{url}}/crypto/orderDetails
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
userID | The userID returned while creating the user |
orderID | ID of the order placed |
Response Data
Parameter | Value | Comments |
---|---|---|
orderID | ID of the order placed | |
status | completed/failed/pending | |
depositToken | Name of token “btc”, ”eth” etc | |
destinationToken | Name of token “btc”, ”eth” etc | |
depositAmount | Deposit token value | |
destinationAmount | Destination token value | |
pair | Token pair used |
Get all Orders
HTTP POST Request
{{url}}/crypto/orders
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
userID | The userID returned while creating the user |
Response Data
Parameter | Value | Comments |
---|---|---|
txns |
|
An array of all the orders placed, each entry will be an object consisting of following details. |
Cancel order
HTTP POST Request
{{url}}/crypto/cancelOrder
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
userID | The userID returned while creating the user |
orderID | ID of the order placed |
Response Data
Parameter | Value |
---|---|
success | true/false |
Wallets
Send
HTTP POST Request
{{url}}/wallets/send
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
userID | The userID returned while creating the user |
token | tokenName |
amount | Amount to withdraw |
receiver | Address to send token to |
Response Data
Parameter | Value | Comments |
---|---|---|
txnID | value | TxnID to track txns |
Get transaction status
HTTP POST Request
{{url}}/wallets/txnStatus
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
userID | The userID returned while creating the user |
txnID | txnID received while trying to send |
Response Data
Parameter | Value | Comments |
---|---|---|
status | success/failed/pending | |
txnHash | transaction hash | txn hash will be sent if success |
Get network fees
To fetch dynamic network fees prevalent at the time of doing the transaction.
HTTP POST Request
{{url}}/wallets/getNetworkFees
Request Parameters
Parameter | Comments |
---|---|
orgID | Your organisation ID (contact [email protected] if you do not have one) |
userID | The userID returned while creating the user |
token | Token to be sent ( e.g eth, btc) |
receiver | Receiver address where token is to be sent |
sender | Vauld platform address from which request is to be initiated |
amount | Amount to be sent in base value ( For 1 ETH 1000000000000000000 needs to be passed |
Response Data
Parameter | Comments |
---|---|
fees | Fees required for the passed variables in base value ( For 1 ETH 1000000000000000000 will be returned) |
Introduction
If you have an already existing wallet, you can use the given API's to enable borrowing for your users.
Organization can request to create an order, add or withdraw collateral and withdraw or payback the loan anytime they like using this partner API
Organization can request to create an order, add or withdraw collateral and withdraw or payback the loan anytime they like using this partner API
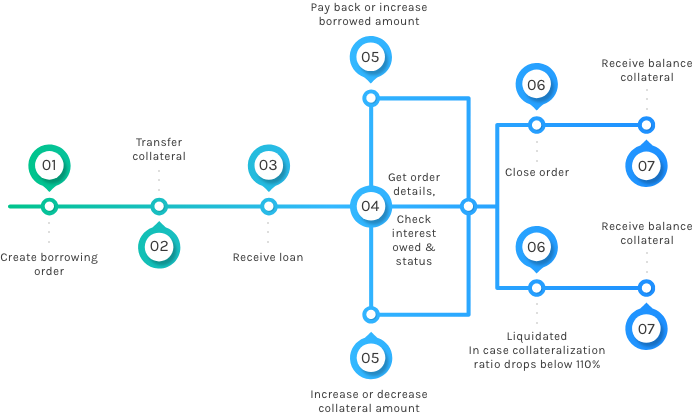
Borrowing Module
Create Order
This endpoint creates a borrowing order for the collateral token.
HTTP Request
POST {{url}}/api/v1/borrowing/createNewOrder
Query Parameters
Parameter | Required | Description |
---|---|---|
orgID | yes | Your OrganisationID |
collateralToken | yes | Collateral token |
loanToken | yes | Token in which you want to receive the loan |
Response Details
Key | Description |
---|---|
success | true or false |
data | Contains order specific details |
collateralDepositAddress | Address at which collateral token needs to be sent to activate the order |
loanRepaymentAddress | Address at which loaned out token needs to be repaid partly or fully |
interestRate | Annual rate of interest for the loan |
orderID | orderID for the borrow order, which can be used for fetching details of the order |
JSON response object format:
{
"success": true,
"data": {
"collateralDepositAddress": "0xce6A74eFaE4a9047Ae63ddb3e94f23e4652606b2",
"loanRepaymentAddress": "0xce6A74eFaE4a9047Ae63ddb3e94f23e4652606b2",
“interestRate” : 8,
"orderID": "5df4a0e31b9f330741a58784"
}
}
Get Borrowing Rates
This endpoint gives out borrowing rates for specified token, if the token parameter is not passed , all supported tokens borrow rates will be returned.
HTTP Request
POST {{url}}/api/v1/borrowing/getRates
URL Parameters
Parameter | Required | Description |
---|---|---|
orgID | yes | Your Organisation ID |
token | yes | Coin for which borrow rates needs to be fetched |
Response Details
Key | Description |
---|---|
success | true or false |
data | Contains coin specific details |
[token] | Token name |
interestRates | Current borrowing interest rate for the fetched token |
JSON response object format:
{
"success": true,
"data": {
"tusd": {
"interestRate": 10
},
"btc": {
"interestRate": 8
},
"dai": {
"interestRate": 10
},
"eth": {
"interestRate": 8
}
}
}
Get order details
This endpoint gives out order details for the created borrowing order.
HTTP Request
POST {{url}}/api/v1/borrowing/getOrderDetails
URL Parameters
Parameter | Required | Description |
---|---|---|
orgID | yes | Your OrganisationID |
orderID | yes | orderID of one of the order created by your organisation |
Response Details
Key | Description |
---|---|
success | true or false |
data | Contains order specific details |
[token] | Token name |
orderID | Order ID of the order |
collateralToken | Current collateral amount, in token base value |
loanToken | Loan token |
loanAmount | Current loan amount that has been loaned out in lieu of collateral token, in token base value |
status | It can be active, liquidated and paid depending on current state of the loan |
collateralRatio | Percentage of Total collateral token value by Total loan value. Less than 150% is unsafe, Below 200% is moderately safe, Above 200% is safe, Automated liquidation at 110%. |
interestRate | Interest rate of loan |
withdrawableCollateral | Collateral that can be withdrawn while maintaining minimum recommended collateral ratio of 150% |
withdrawableLoan | Loan that can be withdrawn while maintaining minimum recommended collateral ratio of 150% |
interest | Pending interest on the loan, in loaned out token |
txns | All transactions on the passed order ID including deposits, repayments and withdrawals. |
JSON response object format:
{
Success:true,
data: {
“orderID”: “5df4a0e31b9f330741a58784”,
“collateralToken”: “eth”,
"collateralAmount": 10000000000000,
"loanToken": "tusd",
"loanAmount": 20000000,
"status": "active",
"collateralRatio": 230,
"interestRate": 8,
"interest": 23879,
“withdrawableCollateral” : 1231749,
“withdrawableLoan”: 1649164
"txns": {
deposits: [
{
date: “2019-12-14T12:45:32.299Z”,
amount: “1000000000000000000”,
txnHash: “0x7c066b62cba0e2527748c175c7570afa36afeb38653288b00db632ce60e9cda3”
},
{
date: “2019-12-14T12:45:32.299Z”,
amount: “1000000000000000000”,
txnHash: “0x7c066b62cba0e2527748c175c7570afa36afeb38653288b00db632ce60e9cda3”
}
],
withdrawals: [
{
date: “2019-12-14T12:45:32.299Z”,
amount: “1000000000000000000”,
txnHash: “0x7c066b62cba0e2527748c175c7570afa36afeb38653288b00db632ce60e9cda3”
},
{
date: “2019-12-14T12:45:32.299Z”,
amount: “1000000000000000000”,
txnHash: “0x7c066b62cba0e2527748c175c7570afa36afeb38653288b00db632ce60e9cda3”
}
],
repayments: [
{
date: “2019-12-14T12:45:32.299Z”,
amount: “1000000000000000000”,
txnHash: “0x7c066b62cba0e2527748c175c7570afa36afeb38653288b00db632ce60e9cda3”
}
],
}
}
Get all Orders
This endpoint gives a brief overview of all the borrowing orders associated with the organisation.
HTTP Request
POST {{url}}/api/v1/borrowing/getAllOrders
URL Parameters
Parameter | Required | Description |
---|---|---|
orgID | yes | Your OrganisationID |
Response Details
Key | Description |
---|---|
success | true or false |
data | Contains order specific details |
orderID | orderID for the borrow order, which can be used for fetching details of the order |
collateralRatio | Percentage of Total collateral token value by Total loan value. Less than 150% is unsafe, Below 200% is moderately safe, Above 200% is safe, Automated liquidation at 110%. |
JSON response object format:
{
"success": true,
"data": [
{
“orderID” : “5df4a0e31b9f330741a58784”,
“collateralRatio”: 130,
},
{
“orderID” : “5df4a0e31b9f330741a58784”,
“collateralRatio”: 230,
},
{
“orderID” : “5df4a0e31b9f330741a58784”,
“collateralRatio”: 111,
},
{
“orderID” : “5df4a0e31b9f330741a58784”,
“collateralRatio”: 190,
}
]
}
Withdraw Collateral
This endpoint enables you to withdraw extra collateral added while maintaining a minimum of 150% collateralization ratio.
HTTP Request
POST {{url}}/api/v1/borrowing/withdrawCollateral
URL Parameters
Parameter | Required | Description |
---|---|---|
orgID | yes | Your OrganisationID |
orderID | yes | OrderID of the order one wishes to withdraw from |
withdrawalAddress | yes | Address where the withdrawn amount needs to be sent |
amount | yes | Amount of token in base value of token associated with the order |
Response Details
Key | Description |
---|---|
success | true or false |
data | Contains order specific details |
token | Coin associated with the order |
orderID | order ID of the order |
withdrawalTxnHash | Transaction hash for the withdrawal transaction |
collateralToken | Collateral token |
collateralAmount | Current collateral amount, in token base value |
loanToken | Loan token |
loanAmount | Current loan amount that has been loaned out in lieu of collateral token, in token base value |
status | It can be active, liquidated and paid depending on current state of the loan |
collateralRatio | Percentage of Total collateral token value by Total loan value. Less than 150% is unsafe, Below 200% is moderately safe, Above 200% is safe, Automated liquidation at 110%. |
interestRate | Interest rate of loan |
withdrawableCollateral | Collateral that can be withdrawn while maintaining minimum recommended collateral ratio of 150% |
withdrawableLoan | Loan that can be withdrawn while maintaining minimum recommended collateral ratio of 150% |
interest | Pending interest on the loan, in loaned out token |
txns | All transactions on the passed order ID including deposits, repayments and withdrawals. |
JSON response object format:
{
Success:true,
data: {
“orderID”: “5df4a0e31b9f330741a58784”,
“withdrawalTxnHash” : “0x7c066g62cba0e2527748c175c7570afa36afeb38653288b00db632ce60e9cda3”,
“collateralToken”: “eth”,
"collateralAmount": 10000000000000,
"loanToken": "tusd",
"loanAmount": 20000000,
"status": "active",
"collateralRatio": 230,
"interestRate": 8,
"interest": 23879,
“withdrawableCollateral” : 1231749,
“withdrawableLoan”: 1649164
"txns": {
deposits: [
{
date: “2019-12-14T12:45:32.299Z”,
amount: “1000000000000000000”,
txnHash: “0x7c066b62cba0e2527748c175c7570afa36afeb38653288b00db632ce60e9cda3”
},
{
date: “2019-12-14T12:45:32.299Z”,
amount: “1000000000000000000”,
txnHash: “0x7c066b62cba0e2527748c175c7570afa36afeb38653288b00db632ce60e9cda3”
}
],
withdrawals: [
{
date: “2019-12-14T12:45:32.299Z”,
amount: “1000000000000000000”,
txnHash: “0x7c066b62cba0e2527748c175c7570afa36afeb38653288b00db632ce60e9cda3”
},
{
date: “2019-12-14T12:45:32.299Z”,
amount: “1000000000000000000”,
txnHash: “0x7c066b62cba0e2527748c175c7570afa36afeb38653288b00db632ce60e9cda3”
}
],
repayments: [
{
date: “2019-12-14T12:45:32.299Z”,
amount: “1000000000000000000”,
txnHash: “0x7c066b62cba0e2527748c175c7570afa36afeb38653288b00db632ce60e9cda3”
}
],
}
}
Withdraw Loan
This endpoint enables you to withdraw loan while maintaining a minimum of 150% collateralization ratio.
HTTP Request
POST {{url}}/api/v1/borrowing/withdrawLoan
URL Parameters
Parameter | Required | Description |
---|---|---|
orgID | yes | Your OrganisationID |
orderID | yes | OrderID of the order one wishes to withdraw from |
withdrawalAddress | yes | Address where the withdrawn amount needs to be sent |
amount | yes | Amount of token in base value of token associated with the order |
Response Details
Key | Description |
---|---|
success | true or false |
data | Contains order specific details |
[token] | Token name |
orderID | order ID of the order |
withdrawalTxnHash | Transaction hash for the withdrawal transaction |
collateralToken | Collateral token |
collateralAmount | Current collateral amount, in token base value |
loanToken | Loan token |
loanAmount | Current loan amount that has been loaned out in lieu of collateral token, in token base value |
status | It can be active, liquidated and paid depending on current state of the loan |
collateralRatio | Percentage of Total collateral token value by Total loan value. Less than 150% is unsafe, Below 200% is moderately safe, Above 200% is safe, Automated liquidation at 110%. |
interestRate | Interest rate of loan |
withdrawableCollateral | Collateral that can be withdrawn while maintaining minimum recommended collateral ratio of 150% |
withdrawableLoan | Loan that can be withdrawn while maintaining minimum recommended collateral ratio of 150% |
interest | Pending interest on the loan, in loaned out token |
txns | All transactions on the passed order ID including deposits, repayments and withdrawals. |
JSON response object format:
{
Success:true,
data: {
“orderID”: “5df4a0e31b9f330741a58784”,
“withdrawalTxnHash” : “0x7c066g62cba0e2527748c175c7570afa36afeb38653288b00db632ce60e9cda3”,
“collateralToken”: “eth”,
"collateralAmount": 10000000000000,
"loanToken": "tusd",
"loanAmount": 20000000,
"status": "active",
"collateralRatio": 230,
"interestRate": 8,
"interest": 23879,
“withdrawableCollateral” : 1231749,
“withdrawableLoan”: 1649164
"txns": {
deposits: [
{
date: “2019-12-14T12:45:32.299Z”,
amount: “1000000000000000000”,
txnHash: “0x7c066b62cba0e2527748c175c7570afa36afeb38653288b00db632ce60e9cda3”
},
{
date: “2019-12-14T12:45:32.299Z”,
amount: “1000000000000000000”,
txnHash: “0x7c066b62cba0e2527748c175c7570afa36afeb38653288b00db632ce60e9cda3”
}
],
withdrawals: [
{
date: “2019-12-14T12:45:32.299Z”,
amount: “1000000000000000000”,
txnHash: “0x7c066b62cba0e2527748c175c7570afa36afeb38653288b00db632ce60e9cda3”
},
{
date: “2019-12-14T12:45:32.299Z”,
amount: “1000000000000000000”,
txnHash: “0x7c066b62cba0e2527748c175c7570afa36afeb38653288b00db632ce60e9cda3”
}
],
repayments: [
{
date: “2019-12-14T12:45:32.299Z”,
amount: “1000000000000000000”,
txnHash: “0x7c066b62cba0e2527748c175c7570afa36afeb38653288b00db632ce60e9cda3”
}
],
}
}
Close Position
This endpoint liquidated enough collateral to pay back the loan and releases the remaining collateral.
HTTP Request
POST {{url}}/api/v1/borrowing/close
URL Parameters
Parameter | Required | Description |
---|---|---|
orgID | yes | Your OrganisationID |
receiverAddress | yes | Address to which remaining collateral should be sent. |
orderID | yes | orderID of one of the order created by your organisation |
Response Details
Key | Description |
---|---|
success | true or false |
data | Contains order specific details |
txnHash | Transaction hash of the transaction which released remaining collateral to receiverAddress |
JSON response object format:
{
"success": true,
"data": {
"txnHash": "0x7c066b62cba0e2527748c175c7570afa36afeb38653288b00db632ce60e9cda3",
}
}
Register Webhook
This endpoint creates registers a URL for a webhook that intimates about change in collateralization ratio.
HTTP Request
POST {{url}}/api/v1/borrowing/registerWebhook
URL Parameters
Parameter | Required | Description |
---|---|---|
orgID | yes | Your OrganisationID |
orderID | yes | orderID of one of the order created by your organisation |
url | yes | URL where change in collateralization ratio should be informed |
Response Details
Key | Description |
---|---|
success | true or false |
JSON response object format:
{
"success": true,
}
Webhook Response
If collateral ratio below 150% and above 125%, webhook is triggered once every 2 days, if it stays the same way,
If collateral ratio drops below 125%, webhook is triggered once a day
If collateral ratio at or below 115%, webhook is triggered every 6hours
if at 110% , webhook is triggered to notify liquidation
If collateral ratio drops below 125%, webhook is triggered once a day
If collateral ratio at or below 115%, webhook is triggered every 6hours
if at 110% , webhook is triggered to notify liquidation
HTTP Request
POST
Add more collateral and pay back loan
For adding more collateral or paying back loan, send the funds to collateralDepositAddress or loanRepaymentAddress respectively.
After confirmation from the blockchain, order and collateralization ratio will be automatically updated
Introduction
If you have an already existing wallet, you can use the given API's to enable earn interest for your user funds.
An organization can create a lending order anytime, move funds to the wallet and withdraw the funds anytime they like.
Interest is calculated daily and paid out every week.
An organization can create a lending order anytime, move funds to the wallet and withdraw the funds anytime they like.
Interest is calculated daily and paid out every week.
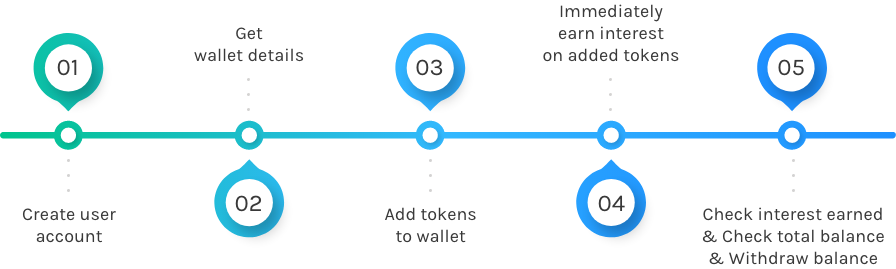
Lending Module
Create Order
This endpoint creates a lending order for the input token.
HTTP Request
POST {{url}}/api/v1/lending/createNewOrder
Query Parameters
Parameter | Required | Description |
---|---|---|
orgID | yes | Your OrganisationID |
token | yes | Coin for which lend order needs to be created |
Response Details
Key | Description |
---|---|
success | true or false |
data | Contains order specific details |
receiverAddress | Address at which token amount needs to be sent to activate the order and start earning interest |
orderID | orderID for the lend order, which can be used for fetching details of the order |
JSON response object format:
{
"success": true,
"data": {
"receiverAddress": "0xce6A74eFaE4a9047Ae63ddb3e94f23e4652606b2",
"orderID": "5df4a0e31b9f330741a58784"
}
}
Get Lending Rates
This endpoint gives out lending rates for specified token, if token parameter is not passed , all supported tokens loan rates will be returned.
HTTP Request
POST {{url}}/api/v1/lending/getRates
URL Parameters
Parameter | Required | Description |
---|---|---|
orgID | yes | Your Organisation ID |
token | yes | Coin for which lend rates needs to be fetched |
Response Details
Key | Description |
---|---|
success | true or false |
data | Contains coin specific details |
[token] | Token name |
interestRates | Current lending interest rate for the fetched token |
JSON response object format:
{
"success": true,
"data": {
"tusd": {
"interestRate": 10
},
"btc": {
"interestRate": 8
},
"dai": {
"interestRate": 10
},
"eth": {
"interestRate": 8
}
}
}
Get order details
This endpoint gives out order details for the created lending order.
HTTP Request
POST {{url}}/api/v1/lending/getOrderDetails
URL Parameters
Parameter | Required | Description |
---|---|---|
orgID | yes | Your OrganisationID |
orderID | yes | orderID of one of the order created by your organisation |
Response Details
Key | Description |
---|---|
success | true or false |
data | Contains order specific details |
[token] | Token name |
currentPrinciple | Current principle for this order in token base value |
interestEarned | Total interest earned for this order in token base value |
interestPaidOut | Total interest paid out for this order |
isOrderActive | True or false, depending upon whether deposit was made to the receiver address |
txns | This contains all the transactions associated with this order that includes deposits, withdrawals and interest payments till date. |
JSON response object format:
{
"success": true,
"data": {
"token” : “eth”,
“currentPrinciple” : “1000000000000000000”,
“interestEarned” : “7000000000000000”,
“interestPaidOut” : “1000000000000000”,
“isOrderActive” : true,
txns: {
deposits: [
{
date: “2019-12-14T12:45:32.299Z”,
amount: “1000000000000000000”,
txnHash: “0x7c066b62cba0e2527748c175c7570afa36afeb38653288b00db632ce60e9cda3”
},
{
date: “2019-12-14T12:45:32.299Z”,
amount: “1000000000000000000”,
txnHash: “0x7c066b62cba0e2527748c175c7570afa36afeb38653288b00db632ce60e9cda3”
}
],
withdrawals: [
{
date: “2019-12-14T12:45:32.299Z”,
amount: “1000000000000000000”,
txnHash: “0x7c066b62cba0e2527748c175c7570afa36afeb38653288b00db632ce60e9cda3”
},
{
date: “2019-12-14T12:45:32.299Z”,
amount: “1000000000000000000”,
txnHash: “0x7c066b62cba0e2527748c175c7570afa36afeb38653288b00db632ce60e9cda3”
}
],
interestPaidOut: [
{
date: “2019-12-14T12:45:32.299Z”,
amount: “1000000000000000000”,
}
],
}
}
Get all Orders
This endpoint gives a brief overview of all the orders associated with the organisation.
HTTP Request
POST {{url}}/api/v1/lending/getAllOrders
URL Parameters
Parameter | Required | Description |
---|---|---|
orgID | yes | Your OrganisationID |
Response Details
Key | Description |
---|---|
success | true or false |
data | Contains order specific details |
orderID | orderID for the lend order, which can be used for fetching details of the order |
token | Coin associated with the order |
currentPrinciple | Current principle for the associated orderID |
JSON response object format:
{
"success": true,
"data": [
{
“orderID” : “5df4a0e31b9f330741a58784”,
“token” : “eth”,
“currentPrinciple” : “10000000000000”
},
{
“orderID” : “5df4a0e31b9f330741a58784”,
“token” : “dai”,
“currentPrinciple” : “1000025250000000”
},
{
“orderID” : “5df4a0e31b9f330741a58784”,
“token” : “tusd”,
“currentPrinciple” : “12346000000”
},
{
“orderID” : “5df4a0e31b9f330741a58784”,
“token” : “btc”,
“currentPrinciple” : “1200000”
}
]
}
Withdrawal
This endpoint enables you to withdraw any amount upto a maximum of current principle associated with the order.
HTTP Request
POST {{url}}/api/v1/lending/withdraw
URL Parameters
Parameter | Required | Description |
---|---|---|
orgID | yes | Your OrganisationID |
orderID | yes | OrderID of the order one wishes to withdraw from |
withdrawalAddress | yes | Address where the withdrawn amount needs to be sent |
amount | yes | Amount of token in base value of token associated with the order |
Response Details
Key | Description |
---|---|
success | true or false |
data | Contains order specific details |
orderID | orderID for the lend order, which can be used for fetching details of the order |
token | Coin associated with the order |
currentPrinciple | Current principle for the associated orderID |
JSON response object format:
{
"success": true,
"data": {
“txnHash: “0x7c066b62cba0e2527748c175c7570afa36afeb38653288b00db632ce60e9cda3”
}
}